Jenkins
This topic explains in detail the Integration between Appdome and Jenkins.
To successfully secure your applications with Appdome from Jenkins platform, you can either use Appdome build-2secure plugin, or use Appdome DEV-API.
build-2secure plugin - Recommended
With Appdome build-2secure plugin, you can easily secure and customize your mobile apps on Jenkins, including signing your app with your own enterprise certificate for added flexibility and control. No coding or technical expertise is required.
For detailed information about integrating build-2secure into your Jenkins job, please follow: build-2secure KB
DEV-API
To successfully use Appdome DEV-API in Jenkins environment, please follow the below steps:
Requirements:
Initial Configurations
• click "New Item"
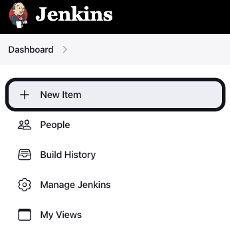
• Give your project a name and choose "Freestyle project" and press "OK":

• On the next page go to "Build Steps"
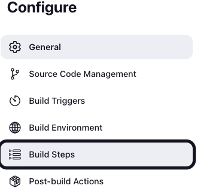
• And click "Add build step" dropdown and there select "Execute shell":
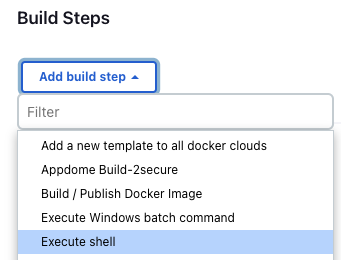
API implementation
Continue with implementing each part of the build process as documented in the How to section.
Example for uploading and building your app:
#!/bin/bash
export APPDOME_API_TOKEN="<YOUR_APPDOME_TOKEN>"
export APP_FILE="<YOUR_APP_FILE>"
export FUSION_SET="<YOUR_FUSION_SET>"
echo "uploading..."
publicLink=$(curl -s --request GET \
--url "https://fusion.appdome.com/api/v1/upload-link" \
--header "Authorization: $APPDOME_API_TOKEN")
curl -s -X PUT "$(echo "$publicLink" | jq -r .url)" \
--header 'Content-Type: application/x-compressed-tar' \
-T $APP_FILE
app=$(curl -s \
--request POST \
--url "https://fusion.appdome.com/api/v1/upload-using-link" \
--header "Authorization: $APPDOME_API_TOKEN" \
--header 'content-type: multipart/form-data' \
--form file_name=$APP_FILE \
--form file_app_id="$(echo "$publicLink" | jq -r .file_id)")
echo "building..."
task_id=$(
curl -s --request POST \
--url "https://fusion.appdome.com/api/v1/tasks" \
--header "Authorization: $APPDOME_API_TOKEN" \
--header 'accept: application/json' \
--header 'content-type: multipart/form-data' \
--form action=fuse \
--form fusion_set_id="$FUSION_SET" \
--form app_id="$(echo "$app" | jq -r .id)" |
jq -r .task_id
)
statusWaiter() {
task_id=$task_id
status="progress"
while [[ $status == "progress" ]]; do
status=$(curl -s --request GET \
--url "https://fusion.appdome.com/api/v1/tasks/$task_id/status?team_id=personal" \
--header 'Content-Type: application/json' \
--header "Authorization: $APPDOME_API_TOKEN" |
jq -r '.status')
sleep 0.5
done
}
statusWaiter "$task_id"
Please replace the placeholders "<YOUR_APPDOME_TOKEN>", "<YOUR_FUSION_SET>" and "<YOUR_APP_FILE>" with the actual values before running the script.
Updated 3 months ago